A major change to AL Language development is here! Collectible errors can hugely improve any validation procedures. And what’s new? Everything is about a new data type ErrorInfo.
ErrorInfo data type
ErrorInfo is a new data type introduced in Microsoft Dynamics 365 Business Central 2021 wave 2 (autumn 2021). This new data type greatly improves error management, user readability of these errors and also enhance information users can get from these errors.
Before this data type, the only way how a developer could raise an error was using the Error(Text) procedure. Once this error was called, the system break the current activity (except if raised inside the Try function, but it’s a different topic).
Now developers have another method Error(ErrorInfo). That means the Error method now accept two different data types as its parameter (Text and also ErrorInfo).
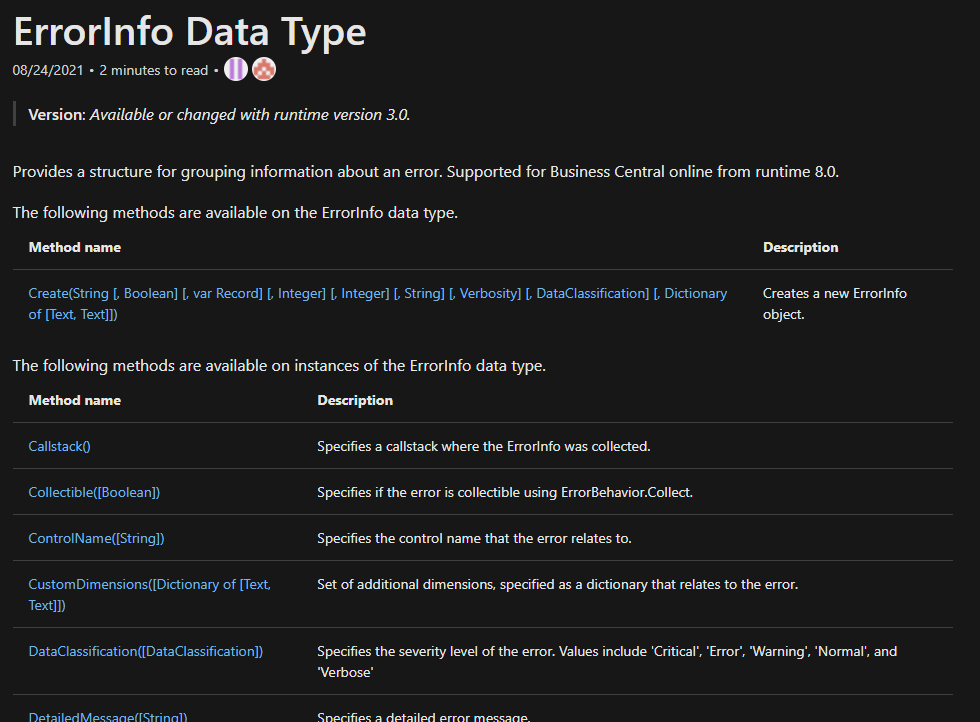
What’s ErrorInfo
ErrorInfo is a complex data type. It contains many methods using which you can enhance the information provided to users or admins. The complete list of all available methods is at Microsoft Doc (https://docs.microsoft.com/en-us/dynamics365/business-central/dev-itpro/developer/methods-auto/errorinfo/errorinfo-data-type).
As a developer, you can use (for the rest of the available methods see Microsoft Docs above):
Message([String])
– Specifies the message that will be sent to telemetry / appear in the client.
DetailedMessage([String])
– Specifies a detailed error message. This message is shown in the client under the “Detailed Information” section, once it is expanded.
CustomDimensions([Dictionary of [Text, Text]])
– You can define Dictionary of Text/Text to show additional information to users. For example, you can add information about the current record or about any calculation.
Verbosity([Verbosity])
– Specifies the severity level of the error. Values include ‘Critical’, ‘Error’, ‘Warning’, ‘Normal’, and ‘Verbose’.
There are other methods (Callstack(), FieldNo([Integer]), Verbosity([Verbosity]), …) which allow defining additional information for users/admins or to read information about the error itself by developers (for example Callstack() returns the whole call stack when the error was raised).
Collectible Errors
Collectible errors are another news that is available with ErrorInfo data type. Using collectible errors we can do more advanced validations and raise the error (or ALL ERRORS) at the end of the whole procedure. This means with only one error visible in the client, users can be notified about all validation errors. In my opinion, this is one of the greatest enhancements of the Business Central usability for many years.
Let’s see some details and examples.
Standard error
The standard error (non-collectible Error(ErrorInfo) or Error(Text)) breaks current activity and show the error message in the client / send it to telemetry. For example, the code below shows an error message containing “Error no. 1”.
local procedure StandardErrorProcedure()
var
Counter: Integer;
begin
for Counter := 1 to 5 do
Error('Error no. %1', Counter);
end;

Collectible Errors
With collectible errors, the system does not break the current activity (even if one or more errors are raised) until the end of the procedure that is defined using ErrorBehavior annotation. Once the method ends and there were errors raised, the error message that contains the first error in the main section and all others in the “Detailed Information” section is raised.
To create a collectible error, the method must be annotated with ErrorBehavior(ErrorBehavior::Collect) syntax and ErrorInfo instances must be set as Collectible(true). See the example below.
[ErrorBehavior(ErrorBehavior::Collect)]
local procedure CollectibleErrorProcedure()
var
MyErrorInfo: ErrorInfo;
Counter: Integer;
begin
for Counter := 1 to 5 do begin
Clear(MyErrorInfo);
MyErrorInfo := ErrorInfo.Create(StrSubstNo('Error no. %1', Counter));
MyErrorInfo.DetailedMessage(StrSubstNo('The error was raised. This is error number %1', Counter));
MyErrorInfo.Collectible(true);
Error(MyErrorInfo);
end;
end;
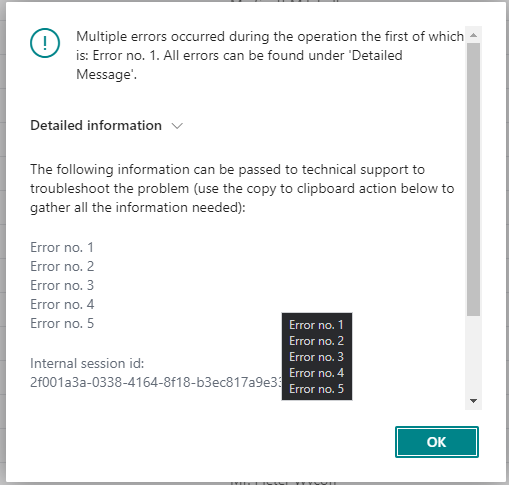
Handling Collectible Errors
You can also handle collectible errors by yourself using three new system methods:
GetCollectedErrors([Boolean]): List of [ ErrorInfo ]
– Using this method you can get a list of all raised Errors marked as collectible. The method accepts a boolean parameter that specifies whether the collected errors should be cleared. If you specify TRUE, it has the same behaviour as calling GetCollectedErrors(false) + ClearCollectedErrors().
HasCollectedErrors(): Boolean
– Returns true if there are any collected errors.
ClearCollectedErrors()
– Clear memory for collected errors. If you clear collected errors, the activity won’t be interrupted at the end of the method and hence no rollback will be performed.