We have already prepared development environment and Android Emulators in previous articles. In this chapter, we will look at how to create a new project and on the generated files.
To start with, open Visual Studio Code (or any other support development environment) and run the command “Flutter: New Project”. Once we have created a new project, we should see something simulator to the picture below.
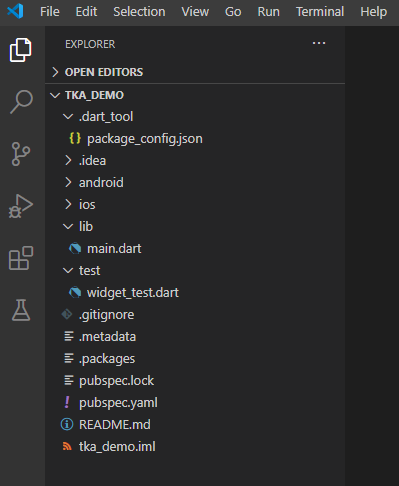
There are many files in the root directory in the newly generated project. Some of the files are generally known (like .gitignore, I will skip them) some are specific to Flutter.
Subdirectories
- android
- This directory contains all the Android related files. The most important things to know is that in this directory (directories), the resources like icons or images are stored.
- From the configuration files, the most important is “AndroidManifest.xml” that specifies everything related just to Android (for example system permissions that to the app requires to run)
- ios
- Similar to the android directory. As these articles are focus on the Android app, I will skip the directory.
- lib
- The main folder where we should write the application code. There are some patterns of how the files should be structured in this directory on which we will look in some of the next articles.
- test
- This folder is used to store and manage testing code for the application.
Files in the root directory
- .metadata
- This file tracks properties of the Flutter project and should not be changed manually.
- .packages
- This file is generated automatically by Pub (Flutter package manager), and like the previous file, it should not be changed manually.
- pubspec.lock
- Similar file to .packages, used by Pub to get the concrete versions for every used package or dependency.
- pubspec.yaml
- This is probably the only file we will sometimes change in the future. The file specifies all flutter packages we have available. If we need to add a new third-party package (for example HTTP), we should do it in this file.
- tka_demo.iml
- Auto-Generated file specifying internal project properties.
main.dart file
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}